The quick start guides you through the steps of adding a FlexPie control to your MVC web application and add data to it.
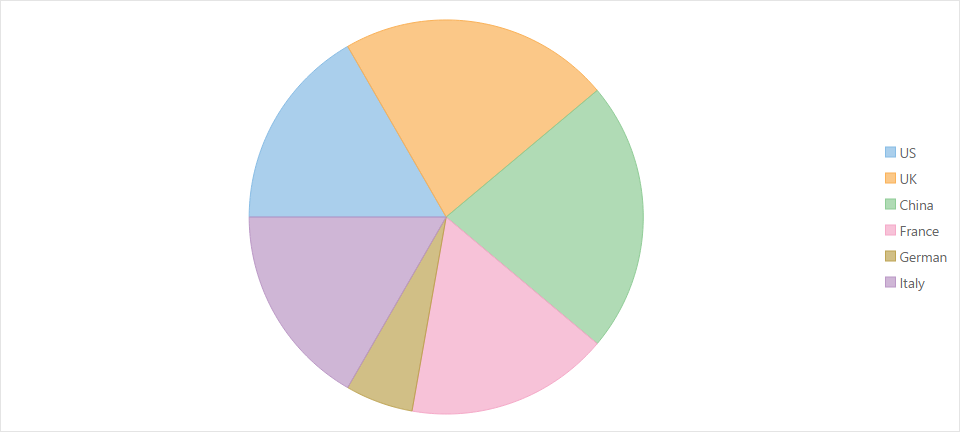
Create an MVC Application
Create a new MVC application using the ComponentOne or VisualStudio templates. For more information about creating an MVC application, see Configuring your MVC Application topic.
Create a Data Source for FlexPie
- Add a new class to the folder Models (for example:
FlexPieDataSource.cs
). See Adding controls to know how to add a new model.
- Add the following code to the new model to define the classes that serve as a datasource for the FlexPie control.
C# |
Copy Code
|
public class FlexPieDataSource
{
public string Country { get; set; }
public int Sales { get; set; }
public static IEnumerable<FlexPieDataSource> GetData()
{
var countries = new[] { "US", "UK", "China", "France", "German", "Italy" };
var rand = new Random(0);
List<FlexPieDataSource> list = new List<FlexPieDataSource>();
for (int i = 0; i < 6; i++)
{
var sales = rand.Next(1, 5);
list.Add(new FlexPieDataSource { Sales = sales, Country = countries[i] });
}
return list;
}
}
|
VB |
Copy Code
|
Public Class FlexPieDataSource
Public Property Country() As String
Get
Return m_Country
End Get
Set
m_Country = Value
End Set
End Property
Private m_Country As String
Public Property Sales() As Integer
Get
Return m_Sales
End Get
Set
m_Sales = Value
End Set
End Property
Private m_Sales As Integer
Public Shared Function GetData() As IEnumerable(Of FlexPieDataSource)
Dim countries = New String() {"US", "UK", "China", "France", "German", "Italy"}
Dim rand = New Random(0)
Dim list As New List(Of FlexPieDataSource)()
For i As Integer = 0 To 5
Dim sales = rand.[Next](1, 5)
list.Add(New FlexPieDataSource() With {
Key.Sales = sales,
Key.Country = countries(i)
})
Next
Return list
End Function
End Class
|
Back to Top
Add a FlexPie control
Complete the following steps to initialize a FlexPie control.
Add a new Controller
- In the Solution Explorer, right click the folder Controllers.
- From the context menu, select Add | Controller. The Add Scaffold dialog appears.
- Complete the following steps in the Add Scaffold dialog:
- Select MVC 5 Controller - Empty template.
- Set name of the controller (for example:
Default1Controller
).
- Click Add.
- Include the MVC references as shown below.
C# |
Copy Code
|
using C1.Web.Mvc;
using C1.Web.Mvc.Serializition;
using C1.Web.Mvc.Chart;
|
- Replace the method
Index()
with the following method.
C# |
Copy Code
|
public ActionResult QuickStart()
{
return View(FlexPieDataSource.GetData());
}
|
VB |
Copy Code
|
Public Function QuickStart() As ActionResult
Return View(FlexPieDataSource.GetData())
End Function
|
Add a View for the Controller
- From the Solution Explorer, expand the folder Controllers and double click the controller
QuickStartController
to open it.
- Place the cursor inside the method
QuickStart()
.
- Right click and select Add View. The Add View dialog appears.
- In the Add View dialog, verify that the view name is QuickStart and View engine is Razor (CSHTML).
- Click Add. A view is added for the controller.
- Instantiate a FlexPie control in the view QuickStart as shown below.
Index.cshtml |
Copy Code
|
@using MvcApplication1.Models
@model IEnumerable<FlexPieDataSource>
@(Html.C1().FlexPie<FlexPieDataSource>()
.Bind("Country", "Sales", Model)
)
|
Index.vbhtml |
Copy Code
|
@ModelType IEnumerable(Of FlexPieDataSource)
@(Html.C1().FlexPie(Of FlexPieDataSource) _
.Bind("Country", "Sales", Model) _
.Height("300px"))
|
Back to Top
Build and Run the Project
- Click Build | Build Solution to build the project.
- Press F5 to run the project.
Append the folder name and view name to the generated URL (for example: http://localhost:1234/
QuickStart/QuickStart) in the address bar of the browser to see the view.
Back to Top