This section describes how to add a C1RadialGauge control to android application and set some of its properties.
This topic comprises of two steps:
The following image shows how the C1RadialGauge appears, after completing the steps above:
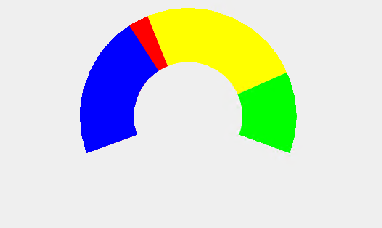
Step 1: Add a C1RadialGauge control
Complete the following steps to initialize a C1RadialGauge control.
In Code
- Add the following reference in the MainActivity class file.
C# |
Copy Code |
using C1.Android.Gauge; |
- Instantiate the C1LinearGauge control in the MainActivity class file and set some of its properties as follows.
C# |
Copy Code |
using Android.App;
using Android.Widget;
using Android.OS;
using C1.Android.Gauge;
namespace AndroidGauge
{
[Activity(Label = "AndroidGauge", MainLauncher = true)]
public class MainActivity : Activity
{
private C1RadialGauge mRadialGauge;
private int mValue = 25;
private int mMin = 0;
private int mMax = 100;
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
mRadialGauge = (C1RadialGauge)FindViewById(Resource.Id.c1RadialGauge1);
mRadialGauge.Enabled = true;
mRadialGauge.Value = mValue;
mRadialGauge.Min = mMin;
mRadialGauge.Max = mMax;
mRadialGauge.Step = 1;
mRadialGauge.ShowText = GaugeShowText.All;
mRadialGauge.IsReadOnly = false;
mRadialGauge.IsAnimated = true;
}
}
} |
- Add the following XML code in the Main.axml in case of C#, to render the control onto the device.
C# |
Copy Code |
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:minWidth="25px"
android:minHeight="25px">
<C1.Android.Gauge.C1RadialGauge
android:minWidth="25px"
android:minHeight="25px"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/c1RadialGauge1" />
</LinearLayout> |
Step 2: Run the Project
In the ToolBar section, select the Android device and then press F5 to view the output.
Back to Top