Posted 10 March 2025, 8:14 am EST
- Updated 11 March 2025, 12:19 am EST
Hi,
You can create a custom command in SpreadJS to remove rows and columns and execute them on button clicks. Please refer to the following code snippet:
var removeSelectedRow = {
canUndo: true,
execute: function (context, options, isUndo) {
var sheet = context.getSheetFromName(options.sheetName);
var Commands = GC.Spread.Sheets.Commands;
if (isUndo) {
Commands.undoTransaction(context, options);
return true;
} else {
Commands.startTransaction(context, options);
var rowIndex = sheet.getActiveRowIndex();
if (rowIndex < 0) return false;
sheet.deleteRows(rowIndex, 1);
Commands.endTransaction(context, options);
return true;
}
}
};
var removeSelectedColumn = {
canUndo: true,
execute: function (context, options, isUndo) {
var sheet = context.getSheetFromName(options.sheetName);
var Commands = GC.Spread.Sheets.Commands;
if (isUndo) {
Commands.undoTransaction(context, options);
return true;
} else {
Commands.startTransaction(context, options);
var colIndex = sheet.getActiveColumnIndex();
if (colIndex < 0) return false;
sheet.deleteColumns(colIndex, 1);
Commands.endTransaction(context, options);
return true;
}
}
};
var commandManager = spread.commandManager();
commandManager.register("removeSelectedRow", removeSelectedRow);
commandManager.register("removeSelectedColumn", removeSelectedColumn);
document.getElementById("removeRowBtn").addEventListener("click", function () {
spread.commandManager().execute({ cmd: "removeSelectedRow", sheetName: spread.getActiveSheet().name() });
});
document.getElementById("removeColBtn").addEventListener("click", function () {
spread.commandManager().execute({ cmd: "removeSelectedColumn", sheetName: spread.getActiveSheet().name() });
});
Observation on Predefined RemoveColumn Command
We have noticed that the predefined RemoveColumn command is not functioning as expected. This issue has been escalated to the development team, and the internal tracking id is SJS-28525.
Regards,
Ankit
remove_row_column_command (3).zip
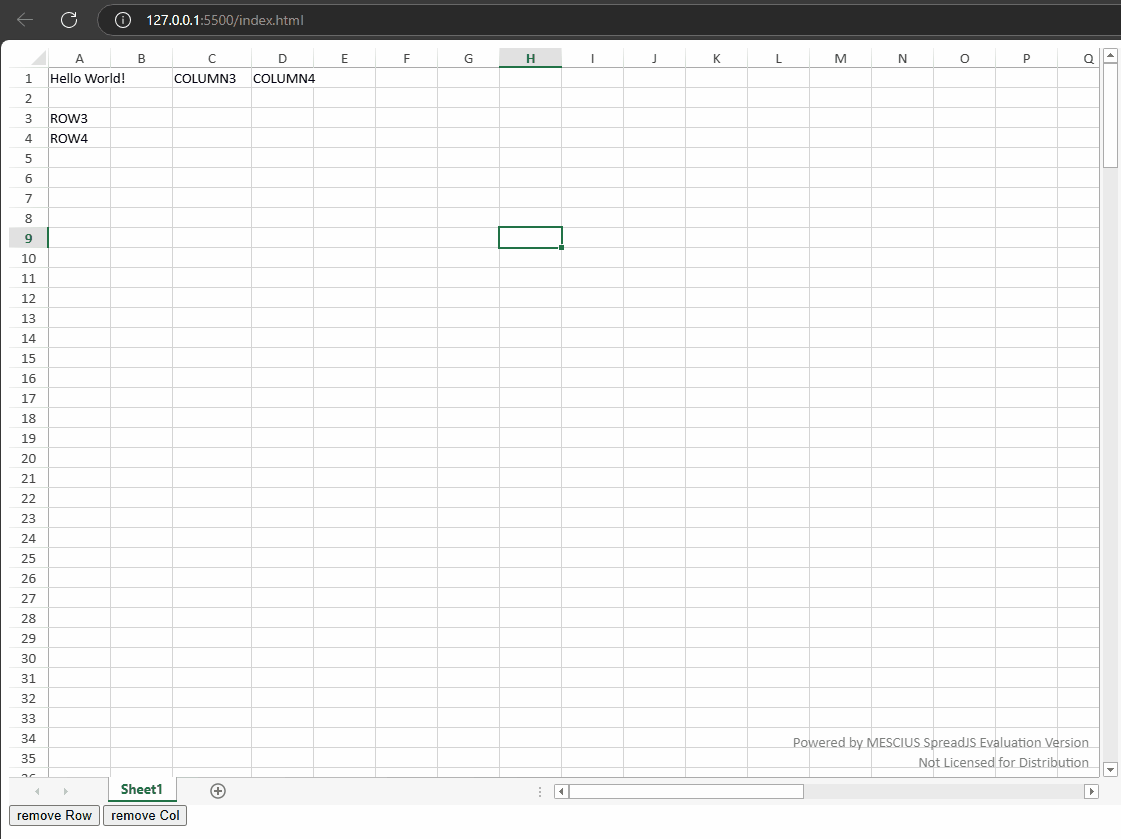