This quick start section gets you started with using Bitmap for loading an image. You begin by creating a WinForms application in Visual Studio, adding a sample image to your application, and adding code to load the sample image in a picture box using Bitmap. The code given in this section illustrates loading an image into bitmap through a stream object.
Complete the steps given below to see how Bitmap can be used to load an image in a picture box.
- Setting up the application and adding a sample image
- Adding code to load image using Bitmap
The following image shows how the application displays an image loaded in bitmap on a button click.
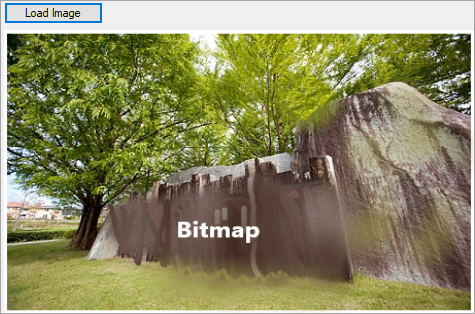
Step 1: Setting up the application and adding a sample image
- Create a WinForms application in Visual Studio.
- Add the following references to your application.
- C1.Win.4
- C1.Win.Bitmap.4.5.2
- C1.Win.C1DX.4.5.2
- In the Solution Explorer, right click your project name and select Add | New Folder and name it as 'Resources'.
- In Visual Studio, add a sample image to the Resources folder and set its Build Action property to Embedded Resource from the Properties pane.
- Add a standard Button control for loading a sample image on button click, and a PictureBox control for displaying the sample image onto the Form.
- Set the Text property of the button to a suitable text from the Properties pane.
- Set the SizeMode property of the picture box to StretchImage from the Properties pane.
Step 2: Adding code to load image using Bitmap
- Switch to the code view and add the following import statements.
Imports C1.Win.Bitmap
Imports C1.Util.DX
Imports System.Reflection
Imports System.IO
using C1.Win.Bitmap;
using C1.Util.DX;
using System.Reflection;
using System.IO;
- Initialize a bitmap in the Form1 class.
'Initialize a Bitmap
Dim bitmap As New C1Bitmap()
//Initialize a Bitmap
C1Bitmap bitmap = new C1Bitmap();
- Subscribe a button click event and add the following code for loading the sample image into bitmap from a stream object.
'Load image through stream on button click
Private Sub Btn_Load_Click(sender As Object, e As EventArgs) Handles Btn_Load.Click
Dim t As Type = Me.GetType
Dim asm As Assembly = t.Assembly
Dim stream As Stream = asm.GetManifestResourceStream(t, "Lenna1.png")
bitmap.Load(stream, New FormatConverter(PixelFormat.Format32bppPBGRA))
UpdateImage()
End Sub
//Load image through stream on button click
private void button1_Click(object sender, EventArgs e)
{
Assembly asm = typeof(Form1).Assembly;
using (Stream stream = asm.GetManifestResourceStream
("LoadBitmapStream.Resources.bitmap-sample.png"))
{
bitmap.Load(stream,
new FormatConverter(PixelFormat.Format32bppPBGRA));
}
UpdateImage();
}
- Add the following code to define UpdateImage method for displaying the image in the picture box.
'Display the image loaded in bitmap
Private Sub UpdateImage()
Dim bmp = pictureBox1.Image
bmp = bitmap.ToGdiBitmap()
pictureBox1.Image = bmp
pictureBox1.Width = bmp.Width
pictureBox1.Height = bmp.Height
End Sub
//Display the image loaded in bitmap
private void UpdateImage()
{
var bmp = pictureBox1.Image as Bitmap;
bmp = bitmap.ToGdiBitmap();
pictureBox1.Image = bmp;
}