In This Topic
The quick start guide familiarizes you with loading an image in Bitmap. You begin with creating a UWP application in Visual Studio, adding C1.UWP.Bitmap reference (dll), Image control, and a button to load an image from stream in C1Bitmap object.
To create a simple UWP application for loading an image in C1Bitmap object, follow these steps:
- Setting up the Application
- Loading an Image into C1Bitmap
The following image shows how the application displays an image loaded from a stream into C1Bitmap:
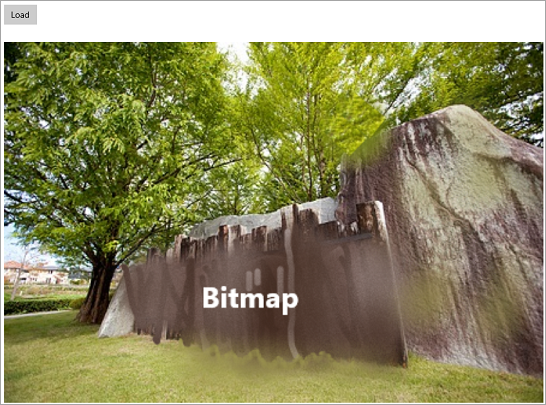
Setting up the Application
To set up the application, follow these steps:
- Create a new project and select Blank App (Universal Windows) in Visual Studio.
- Add the following references to the application.
- In the Solution Explorer, right click your project name and select Add | New Folder. Name the newly added folder. In our case, we have named the new folder as Resources.
- Add a sample image to the Resources folder and set its Build Action property to Embedded Resource from the Properties window.
- Add a standard Button control for loading a sample image and Image control, named img, for displaying the sample image.
- Set the Content property of the button as Load Image.
Back to Top
Loading an Image into C1Bitmap
To load the image in C1Bitmap, follow these steps:
- Switch to the code view and add the following import statements in the code.
- Create the following class objects.
- Add the following code in the class constructor to initialize bitmap and SoftwareBitmapSource.
- Add the following code to the btnLoad_Click event to load the image in C1Bitmap using stream:
Note: To call an asynchronous method on an event, we have used async keyword and await operator.
- Create a method, named UpdateImageSource, to create a SoftwareBitmap, set the source SoftwareBitmap and assign it to the image source:
Back to Top
See Also