Applying Conditional Formatting
In This Topic
C1FlexSheet allows you to change the appearance of an individual cell or a range of cells using conditional formatting. Conditional formatting can be used to compare different data and highlight important data.
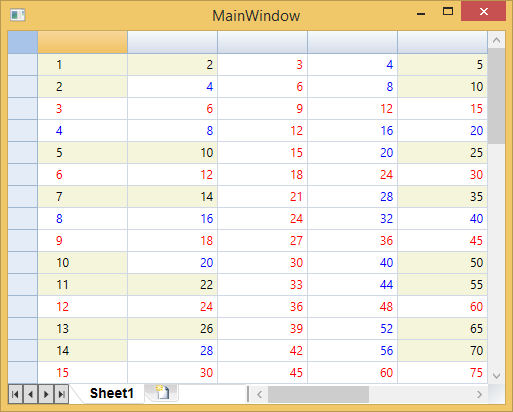
In the above image, you see that data is formatted with different font color and background color depending on some conditions. The numbers divisible by 3 appear red, numbers divisible by 4 appear blue, and the numbers which are neither divisible by 3 nor 4 appear with beige background color.
The following code illustrates the conditions that we have set to change the appearance of data in cells:
Public Class ConditionalCellFactory
Inherits CellFactory
Public Overrides Sub ApplyCellStyles(grid As C1FlexGrid, cellType As CellType,
rng As CellRange, bdr As Border)
MyBase.ApplyCellStyles(grid, cellType, rng, bdr)
If cellType = cellType.Cell Then
Dim tb = If(TypeOf bdr.Child Is TextBlock, TryCast(bdr.Child, TextBlock),
TryCast(DirectCast(bdr.Child, System.Windows.Controls.Grid).Children(1), TextBlock))
If grid(rng.Row, rng.Column) IsNot Nothing Then
If CDbl(grid(rng.Row, rng.Column)) Mod 3 = 0 Then
tb.Foreground = New SolidColorBrush(Colors.Red)
ElseIf CDbl(grid(rng.Row, rng.Column)) Mod 4 = 0 Then
tb.Foreground = New SolidColorBrush(Colors.Blue)
Else
bdr.Background = New SolidColorBrush(Colors.Beige)
End If
End If
End If
End Sub
End Class
public class ConditionalCellFactory : CellFactory
{
public override void ApplyCellStyles(C1FlexGrid grid, CellType cellType,
CellRange rng, Border bdr)
{
base.ApplyCellStyles(grid, cellType, rng, bdr);
if (cellType == CellType.Cell)
{
var tb = bdr.Child is TextBlock ? bdr.Child as TextBlock : _
((System.Windows.Controls.Grid)(bdr.Child)).Children[1] as TextBlock;
if (grid[rng.Row, rng.Column] != null)
{
if ((double)grid[rng.Row, rng.Column] % 3 == 0)
{
tb.Foreground = new SolidColorBrush(Colors.Red);
}
else if ((double)grid[rng.Row, rng.Column] % 4 == 0)
{
tb.Foreground = new SolidColorBrush(Colors.Blue);
}
else
{
bdr.Background = new SolidColorBrush(Colors.Beige);
}
}
}
}
}
In the above code, a class named ConditionalCellFactory is used which inherits from CellFactory class of C1FlexGrid control. The ConditionalCellFactory class contains all the implementation of conditional formatting that we have set.