In This Topic
Adding graphics enhances the appearance of a document and make them visually appealing. You can add various types of shapes in your documents such as, Arc, Bezier, Ellipse, Line, Pie, polygon, PolygonLine, and Rectangle. Use the following code to add graphics such as lines, rectangles, and beziers:
' create document
Dim c1Word As New C1WordDocument()
c1Word.Info.Title = "Graphics primitives sample"
Dim sf As New StringFormat()
sf.Alignment = StringAlignment.Center
sf.LineAlignment = StringAlignment.Center
Dim rc = New RectangleF(250, 100, 150, 20)
Dim font As New Font("Arial", 14, FontStyle.Italic)
c1Word.DrawString(c1Word.Info.Title, font, Color.DeepPink, rc, sf)
c1Word.DrawLine(Pens.Green, 200, 190, 400, 190)
rc = New RectangleF(150, 150, 190, 80)
Using pen As New Pen(Brushes.Blue, 5F)
c1Word.DrawRectangle(pen, rc)
End Using
c1Word.FillRectangle(Color.Gold, rc)
c1Word.ShapeFillOpacity(50)
c1Word.ShapeRotation(25)
rc = New RectangleF(300, 150, 80, 80)
c1Word.DrawEllipse(Pens.Red, rc)
c1Word.FillEllipse(Color.Pink, rc)
c1Word.ShapeFillOpacity(70)
Dim pts As PointF() = New PointF(3) {}
pts(0) = New PointF(200, 200)
pts(1) = New PointF(250, 300)
pts(2) = New PointF(330, 250)
pts(3) = New PointF(340, 140)
c1Word.DrawPolyline(Pens.BlueViolet, pts)
sf = New StringFormat()
sf.Alignment = StringAlignment.Center
sf.LineAlignment = StringAlignment.Far
sf.FormatFlags = sf.FormatFlags Or StringFormatFlags.DirectionVertical
rc = New RectangleF(450, 150, 25, 75)
font = New Font("Verdana", 12, FontStyle.Bold)
c1Word.DrawString("Vertical", font, Color.Black, rc, sf)
pts = New PointF(3) {}
pts(0) = New PointF(372, 174)
pts(1) = New PointF(325, 174)
pts(2) = New PointF(325, 281)
pts(3) = New PointF(269, 281)
c1Word.DrawBeziers(Pens.HotPink, pts)
Dim Sdlg As New SaveFileDialog()
Sdlg.FileName = "document"
Sdlg.Filter = "RTF files (*.rtf)|*.rtf|DOCX (*.docx)|*.docx"
Sdlg.ShowDialog()
c1Word.Save(Sdlg.FileName)
MessageBox.Show("Word document is saved successfully.")
// create document
C1WordDocument c1Word = new C1WordDocument();
c1Word.Info.Title = "Graphics primitives sample";
StringFormat sf = new StringFormat();
sf.Alignment = StringAlignment.Center;
sf.LineAlignment = StringAlignment.Center;
var rc = new RectangleF(250, 100, 150, 20);
Font font = new Font("Arial", 14, FontStyle.Italic);
c1Word.DrawString(c1Word.Info.Title, font, Color.DeepPink, rc, sf);
c1Word.DrawLine(Pens.Green, 200, 190, 400, 190);
rc = new RectangleF(150, 150, 190, 80);
using(Pen pen = new Pen(Brushes.Blue, 5.0 f)) {
c1Word.DrawRectangle(pen, rc);
}
c1Word.FillRectangle(Color.Gold, rc);
c1Word.ShapeFillOpacity(50);
c1Word.ShapeRotation(25);
rc = new RectangleF(300, 150, 80, 80);
c1Word.DrawEllipse(Pens.Red, rc);
c1Word.FillEllipse(Color.Pink, rc);
c1Word.ShapeFillOpacity(70);
PointF[] pts = new PointF[4];
pts[0] = new PointF(200, 200);
pts[1] = new PointF(250, 300);
pts[2] = new PointF(330, 250);
pts[3] = new PointF(340, 140);
c1Word.DrawPolyline(Pens.BlueViolet, pts);
sf = new StringFormat();
sf.Alignment = StringAlignment.Center;
sf.LineAlignment = StringAlignment.Far;
sf.FormatFlags |= StringFormatFlags.DirectionVertical;
rc = new RectangleF(450, 150, 25, 75);
font = new Font("Verdana", 12, FontStyle.Bold);
c1Word.DrawString("Vertical", font, Color.Black, rc, sf);
pts = new PointF[4];
pts[0] = new PointF(372, 174);
pts[1] = new PointF(325, 174);
pts[2] = new PointF(325, 281);
pts[3] = new PointF(269, 281);
c1Word.DrawBeziers(Pens.HotPink, pts);
SaveFileDialog Sdlg = new SaveFileDialog();
Sdlg.FileName = "document";
Sdlg.Filter = "RTF files (*.rtf)|*.rtf|DOCX (*.docx)|*.docx";
Sdlg.ShowDialog();
c1Word.Save(Sdlg.FileName);
MessageBox.Show("Word document is saved successfully.");
In the above code, DrawLine, DrawRectangle, DrawEllipse, DrawPolyline, and DrawBeziers methods are used to draw graphics of different types such as, line, rectangle, bezier, and ellipse.
The document will look similar to the image below:
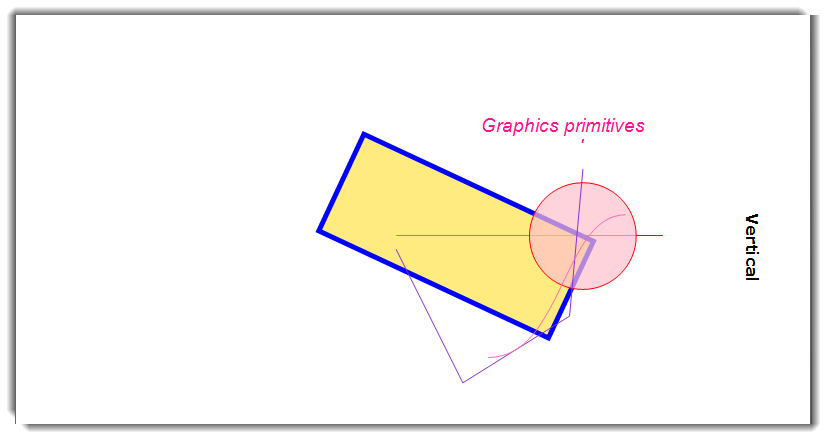